API examples
Examples of how to use the API
-
Show the feedback widget after a user leaves the browser window - exit-intent
-
How to set a project to show as a feedback button and auto-popup on different pages?
-
How to have feedback items pre-tagged according to certain criteria?
Set a language via the API
Set the language when you initiate the Usersnap code snippet (locale: 'de').
Here are the official codes for each language (ISO-639-1-Codes).
(except for traditional (zh-TW) vs simplified (zh-CN) Chinese:
Place the correct language code into the "locale" variable:
<script>
window.onUsersnapCXLoad = function(api) {
api.init({ locale: 'de' })
window.Usersnap = api;
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL_API_KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
Triggering a Usersnap event - example
You can trigger widgets to be opened if a certain Usersnap event is fired. This example shows you how to trigger a Usersnap event.
Setup a widget to trigger when a specific Usersnap event is logged:
Go to your project's "Configuration" page -> "Targeting" tab.
Look for "Trigger & frequency" section of "Targeting" tab. For "Select how you would like to activate this widget" choose "API event".
In "Event" field enter your desired event name e.g. my-awesome-event (do not use quotes) and make sure to hit the "Save" button on the bottom of the page.
Your project needs to be set live (top right corner of page).
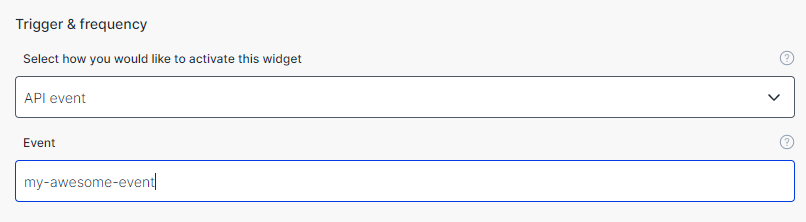
To trigger the widget when the event is logged, use following code example:
// step 1: initialize the global snippet and make Usersnap API available
window.onUsersnapCXLoad = function(api) {
api.init();
// step 2: trigger the widget using a logged event
api.logEvent("my-awesome-event");
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/YOUR-GLOBAL-APIKEY?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
More related info here.
This is a gated featurePlease contact our support for more details. customer success team
Set variables when the widget is opened (button clicked)
You have to install the Usersnap snippet code before. And don't forget to pass on the API to the global variable window.Usersnap for this example.
If you want to pass certain information to all your projects, once the widget is opened, you can add the code like this.
widgetApi.on('open', (event) => {
console.log('setting value now!');
event.api.setValue('visitor', '[email protected]');
event.api.setValue('custom', { userId: '123', environment: 'staging' });
event.api.setValue('labels', 'bug');
event.api.setValue('assignee', '[email protected]');
alert('now, values are set');
});
If you want to pass certain information only to a certain project, once the widget is opened, you can add the code like this.
window.Usersnap.on('open', (event) => {
if (event.apiKey === '[Project-API-key]') {
console.log('setting value now!')
event.api.setValue('visitor', '[email protected]')
event.api.setValue('custom', { userId: '123', environment: 'staging' })
alert('now, values are set')
}
})
Open a feedback widget after a flow/process is finished
Let's assume you have a new flow in your application. After the process or flow is finished, your development team should just fire an Usersnap event and you can display a widget for it.
In the "Targeting" tab under the section of "Trigger & frequency" of your project (in the dashboard), set the activation to "API event". Add the name of the event. Here it's "new_payment_is_finished".
Eh voila, the feedback widget pops up after the process is finished.
<script>
window.Usersnap.logEvent('new_payment_is_finished');
</script>
Using the full page collector for sending feedback requests via email
The easiest way to do that is our newly-released feature, we call it tenderly full page collector.
Using the Usersnap API to open a widget via a link in an email
However, if you feel a bit techier today, you can send a link to a page on your website and initiate a widget via Auto-Popup e.g. https://www.demowebsite.com/?emailtrigger=true ... One of our co-founders thinks he's a developer and build this example for you. 😀
The variable "emailtrigger=true" is only to avoid that the widget popups up on your start page, you only want to show it when your users are clicking on the link in your email. You define in the "Configuration" section in the "Display" tab that this widget should only be shown if the URL contains "?emailtrigger=true".
How to set a project to show as a feedback button and auto-popup on different pages?
Please first select the project's "Activation" to "Button" with "Audience" set to "Nobody".
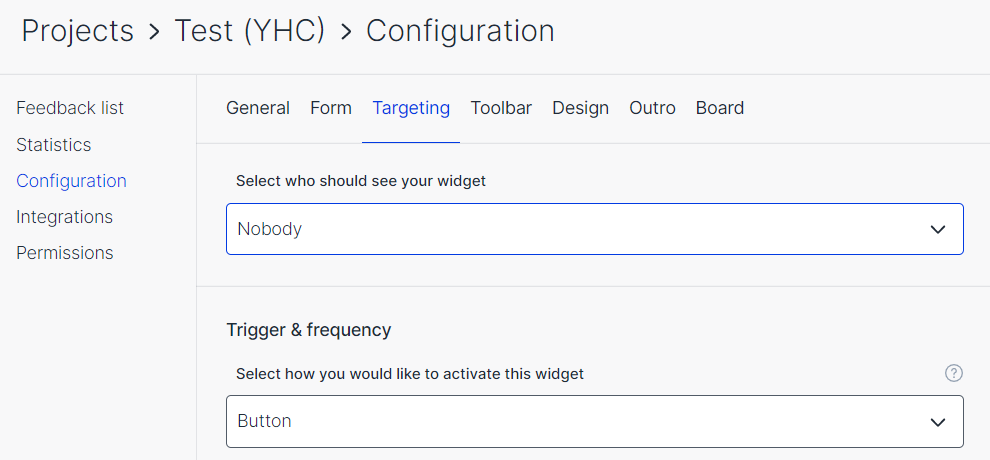
And then please use the following snippet:
window.onUsersnapCXLoad = function (api) {
api.init();
window.Usersnap = api
// to show the button for the widget
window.Usersnap.show('[Project-Api-Key]')
// to show the widget
window.Usersnap
.show('[Project-Api-Key]', { button: null })
.then(widgetApi => widgetApi.open())
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[Global-Api-Key]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
How to pop widgets to different audiences?
If you have 2 groups of users who should see different widgets, please kindly take the following steps.
- Create 2 projects, one for each group of users (for example, one for internal users, whilst the other one for external users)
- Set "Audience" of both projects to "Nobody".
- Use our global snippet with the following code:
<script>
window.onUsersnapCXLoad = function(api) {
api.init();
// showWidgetForInternalUsers variable should be defined before
const projectApiKey = showWidgetForInternalUsers()
? 'PROJECT-API-KEY-FOR-INTERNAL-USERS'
: 'PROJECT-API-KEY-FOR-EXTERNAL-USERS';
api.show(projectApiKey).then(widgetApi => widgetApi.open());
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL-API-KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
More information about API for this particular case can be found here.
Please note that the above snippet is using "showWidgetForInternalUsers" function which identifies whether the widget should be shown to internal users. You can use your own function as well but in any case it should be defined before the code snippet, somewhere on the page done on your end.
Updated 2 months ago