For website or web application
This is a technical documentation of the API
For more user-case oriented examples see Website widget: API examples.
What's mentioned below ONLY works with the space snippet, i.e. the global API key.
Installation
window.onUsersnapLoad = function(api) {
api.init();
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/<GLOBAL_API_KEY>?onload=onUsersnapLoad';
document.getElementsByTagName('head')[0].appendChild(script);
API object
interface GlobalApi {
init: (options: InitOptions) => Promise<void>,
destroy: () => Promise<void>,
logEvent: (eventName: string) => Promise<void>,
on: (eventName: string, callback: (event: any) => void) => void,
off: (eventName: string, callback: (event: any) => void) => void,
}
init()
api.init({
custom: {
applicationLanguage : "de",
},
user: {
userId: "123",
email: "[email protected]",
},
locale: 'de',
});
The init function initializes the global api. It must be called before calling any other api method.
Params
options
options.custom: Record<string, any>
– An object holding custom data. This data will be passed along with every feedbackoptions.user
– An object holding information about the current useroptions.user.email: string
– Will be pre-filled in the widget and attached to every feedbackoptions.user.userId: string
– Can be used for determining whether to show a widget or notoptions.locale: string
– Language in which widgets should be displayedoptions.useSystemFonts: boolean
– Can be used to disable loading external fonts. Defaults tofalse
options.useLocalStorage: boolean
– Can be used to disable usage of localStorage. Defaults totrue
options.nativeScreenshot: boolean
– Can be used to enable the usage of the native screenshot feature. Defaults tofalse
options.collectGeoLocation: 'all' | 'none'
– can be used to disable the collection of IP and geo location. Defaults to'all'
Returns Promise<void>
Promise<void>
destroy()
api.destroy()
Destroys the global api and removes all widgets from the DOM of the webpage.
After calling destroy()
it is necessary to call init()
before calling any other method.
Returns Promise<void>
Promise<void>
logEvent()
api.logEvent('my-event') // will open a widget if one was configured for this event
Can be used to trigger widgets by specific event names.
Params
eventName: string
– the name of the event
Returns Promise<void>
Promise<void>
on()
function handleSubmit() {
console.log('Widget was submitted')
}
api.on('submit', handleSubmit)
Registers an event handler for widget events.
For a detailed list of available events and how they can be used see section Widget Events
Params
eventName: string
– the name of the widget event you want to listen tocallback: (event: WidgetEvent) => void
– callback to handle the event. The structure of the received object depends on the event.
Returns void
void
off()
api.off('submit', handleSubmit)
Removes an event handler
Params
eventName: string
– the name of the widget event want to remove the listener fromcallback: (event: WidgetEvent) => void
– same callback that was used for registering the event
Returns void
void
Widget Events
open
– A widget was opened.close
– A widget was closedbeforeSubmit
– Before form values are submittedsubmit
– After form values were submitted
open
event
open
eventtype ValueKey = 'assignee' | 'custom' | 'labels' | 'visitor'
interface OpenEvent {
apiKey: string,
api: {
setValue: (key: ValueKey, value: any) => void,
},
}
apiKey
– API key of the widget that was openedapi.setValue
– method to set form values
Please note that for the open
event, you should use the "API event" for triggering the widget in the activation settings of your project:
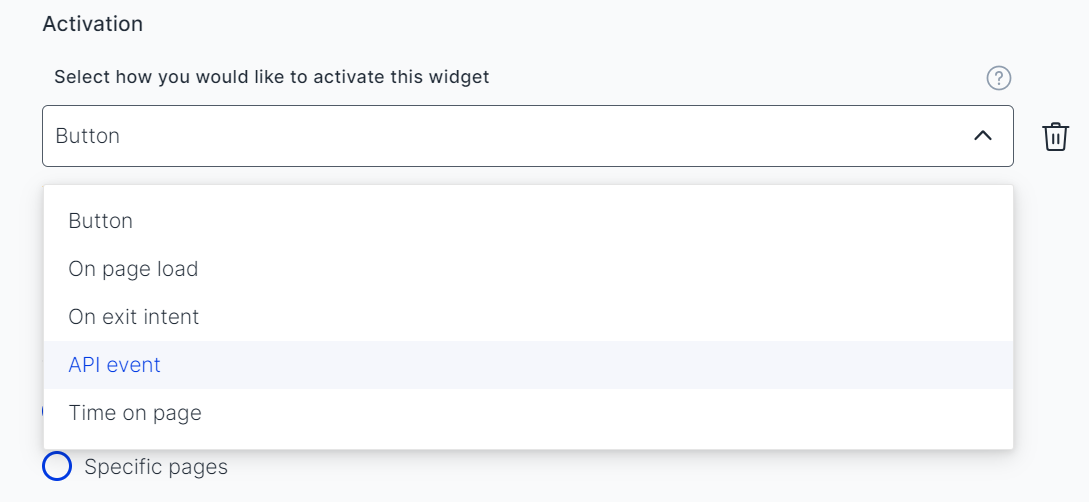
Example
function handleWidgetOpened(event) {
// You can check whether a specific widget was called
if (event.apiKey !== '<PROJECT_API_KEY>') return;
// Set Assignee. A User with this email has to exist in your Usersnap account
event.api.setValue('assignee', '[email protected]')
// Set custom data
event.api.setValue('custom', { applicationLanguage : 'de' })
// Set labels
event.api.setValue('labels', ['bug', 'critical'])
// Set email
event.api.setValue('visitor', '[email protected]')
}
api.on('open', handleWidgetOpened)
close
event
close
eventinterface CloseEvent {
apiKey: string,
isCancel: boolean,
}
apiKey
– API key of the widget that was closedisCancel
– istrue
in case the widget was closed before submitting a feedback item
Example
function handleWidgetClosed(event) {
if (event.isCancel) {
// do something
}
}
api.on('close', handleWidgetClosed)
beforeSubmit
event
beforeSubmit
eventtype ValueKey = 'assignee' | 'custom' | 'labels' | 'visitor'
interface BeforeSubmitEvent {
apiKey: string,
api: {
setValue (key: ValueKey, value: any) => void,
}
values: {
assignee?: string,
custom?: any,
labels?: string,
visitor: string,
}
}
apiKey
– API key of the widget that will be submittedapi.setValue
– method to set form values. Allows changing values before they are submitted.values
– values that will be submitted
Example
function handleBeforeSubmit(event) {
var labels = event.values.labels
if (labels && labels.includes('bug')) {
event.api.setValue('assignee', '[email protected]')
}
}
api.on('beforeSubmit', handleBeforeSubmit)
submit
event
submit
eventinterface CloseEvent {
apiKey: string,
}
apiKey
– API key of the widget that was submitted
Example
function handleSubmit() {
// do something
}
api.on('submit', handleSubmit)