Usersnap API
Usersnap snippet code (global snippet) REQUIRED
Please, be aware that this API needs the standard Usersnap snippet code (the global snippet). This snippet is a code you or your developer have to install once and then, you can decide which feedback widget should be displayed on what pages. The following API examples are ONLY for the standard Usersnap snippet installations.
Using API methods
If you have further questions on the installation and how to use the global API-key, go here. If you are using a well-known framework for your web application, read this section
Initializing the API
The init()
function initializes the API. It must be called before calling any other API method.
init()
can be used to set default values that will be submitted to Usersnap, as follows:
Setting default values when initializing the widget
api.init({
custom: {
applicationLanguage : "de",
},
user: {
userId: "123",
email: "[email protected]",
},
});
"custom"
: Any custom data that should be passed along with the feedback"user"
: User data from known users (can include email or userId)user.email
: the email of the user. Is used to determine whether to show a widget or not.user.userId
: the id of the user. Is used to determine whether to show a widget or not.
The custom data will show up on your Usersnap dashboard as follows:
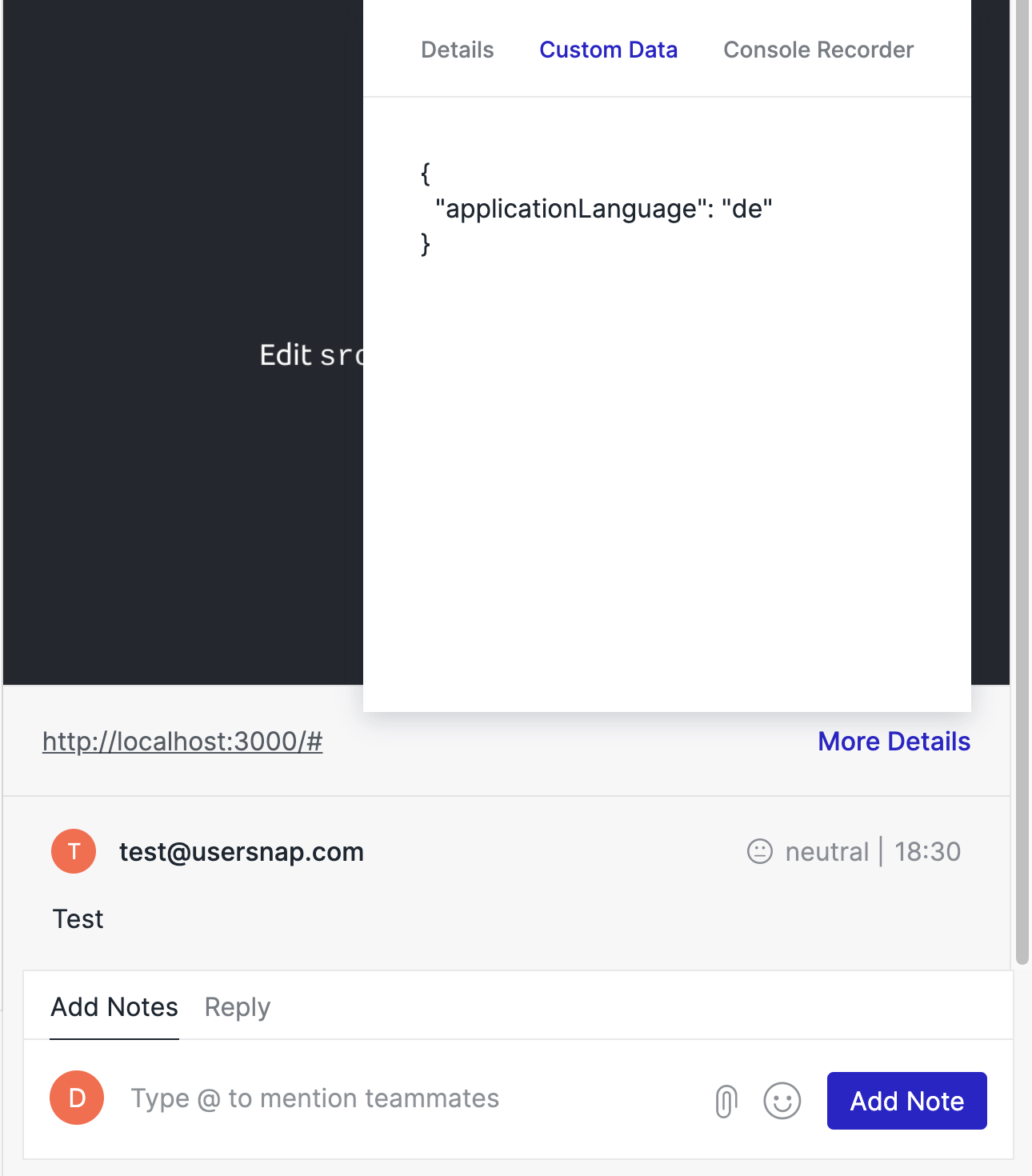
Passing custom data with Embed widget
It is possible to pass custom data via Embed the rating widget into an email .
Please use custom URL parameter with an encoded JSON valid value.
For example, https://collector.usersnap.com/[PROJECT-API-KEY]?custom={%22someUserAttribute%22:12345}
"label"
and "assignee"
: The labels and assignees can be set dynamically behind the scene when the widget is initiated.
Basically, this API grants you the option to have the values set even when the fields are hidden.
These events are available via the API
Events are special behavior in the code that is calling the widgets to open, close, etc. From now on the API events are available for both: project-specific widgets and global snippet widgets. Additionally, it also works for widgets that open other widgets and you can differentiate between those.
-
load – The widget was loaded (initialized)
-
destroy – The widget was destroyed
-
open – The widget was opened
-
closing - Before the widget is closed
-
close – The widget was closed (after closed)
-
submit – The widget’s form was submitted
These events should not be mixed up with the Usersnap event that can be fired in case you want to pop up a widget after a user's action (AutoPopup).
How to use events in your Usersnap snippet code
Some examples of how you can use the Usersnap API events.
<script>
window.onUsersnapCXLoad = function(api) {
api.init({ locale: 'de' })
window.Usersnap = api;
function setVisitorOnOpen(event) {
// in case you have only one widget the value will be set on this widget
event.api.setValue('visitor', '[email protected]');
}
api.on('open', setVisitorOnOpen);
setTimeout(() => {
// you can also remove any event listener at any moment
api.off('open', setVisitorOnOpen);
}, 10000)
api.on('open', function(event) {
// in case you have multiple widgets you can filter them by "apiKey" property
if (event.apiKey === '<Project-specific API-key>') {
event.api.setValue('visitor', '[email protected]');
}
// if you use project-specific widget which opens other widgets,
// the event for this "top" widget will have empty "apiKey"
if (!event.apiKey) {
event.api.setValue('visitor', '[email protected]');
}
});
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL_API_KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
Show a feedback button
Shows the feedback button. Calling this method disables the display rules for this widget.
PROJECT_API_KEY
: the API key of the project (not the global API-key of the account)
api.show('[PROJECT_API_KEY]')
Hide a feedback button
Hides the feedback button. Calling this method disables the display rules for this widget.
PROJECT_API_KEY
: the API key of the project (not the global API-key of the account)
api.hide('[PROJECT_API_KEY]')
The feedback button of a certain project can be hidden/displayed independently via the API.
Just call those methods and pass the APIKEY of the according project.
Open a feedback widget
PROJECT_API_KEY
: the API key of the project (not the global API-key of the account)
Displays a widget via the API.
api.show('[PROJECT_API_KEY]'').then((widgetApi) => widgetApi.open())
Hide a feedback widget
Hides a widget via the API.
api.hide('[PROJECT_API_KEY]'').then((widgetApi) => widgetApi.open())
Destroy the API
Destroys the global API. You need to call init again before calling any other method.
api.destroy()
Passing user information via the API
You can pass user information to Usersnap to increase the options to target specific user groups and provide targeted experiences.
// assuming a global widget config looking something like this
window.onUsersnapCXLoad = function(api) {
api.init({
user: {
email: "[email protected]", // email address is pre-set
userId: "USER_ID", // UserID defines that a user is "logged" for Usersnap
}
});
}
Trigger a Usersnap event
Triggers a Usersnap event that can be used to display the widget. It evaluates the display rules taking the last event into account.
eventName
: the event name (string)
Triggering an Usersnap event - example
More related info here.
How to show the button only on desktop or mobile screens?
Here's a little custom coding to show your feedback button only on desktop screens. Or you can use a similar if-clause to only show the feedback button on mobile devices. If you have any questions, please contact our lovely customer success team.
<script>
// this will only be shown on desktop. You can also use mobile or tablet here
if ( testScreen() == 'desktop' ) {
window.onUsersnapCXLoad = function(api) {
api.init();
window.Usersnap = api;
// feedback project for desktop screens
window.Usersnap.show('INSERT-API-KEY-OF-THIS-PROJECT');
console.log('desktop menu widget');
}
var script = document.createElement('script');
script.defer = 1;
// for the installation of Usersnap
script.src = 'https://widget.usersnap.com/global/load/INSERT-GLOBAL-API-KEY?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
}
function testScreen() {
var S_width = window.innerWidth;
var screenType;
if (S_width <= 520) {
screenType = "mobile";
} else if (S_width <= 820) {
screenType = "tablet";
} else {
screenType = "desktop";
}
return screenType;
}
</script>
Installation
Please, go to the Installation section.
Remove tracking of the location
If you don't want Usersnap to track the location (country, city) of the users, you can deactivate this by initiating the snippet code with this parameter.
- collectGeoLocation: 'none'
<script>
window.onUsersnapCXLoad = function(api) {
api.init({
collectGeoLocation: 'none'
});
window.Usersnap = api;
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL_API_KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
For any further questions, please contact our lovely customer success team.
Deactivate using localStorage (Cookies)
The Usersnap feedback widgets do not use cookies, but we use the localStorage to store a few parameters. From a data privacy standpoint (GDPR), the localStorage is seen similar to cookies. If you want to deactivate the localStorage for your website or web application, you can do this with the following parameters.
Please, be aware that this has a few disadvantages for your users. For example, the email is not remembered for the next time, they give feedback. Also, the assignees are not stored.
<script>
window.onUsersnapCXLoad = function(api) {
api.init({
useLocalStorage: false
});
window.Usersnap = api;
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL_API_KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
Deactivate GoogleFonts in the feedback widgets
The Usersnap feedback widgets use GoogleFonts to make the widgets look nicer. If you don't want to use GoogleFonts, you can deactivate them with the following attribute.
<script>
window.onUsersnapCXLoad = function(api) {
api.init({
useSystemFonts: false
});
window.Usersnap = api;
}
var script = document.createElement('script');
script.defer = 1;
script.src = 'https://widget.usersnap.com/global/load/[GLOBAL_API_KEY]?onload=onUsersnapCXLoad';
document.getElementsByTagName('head')[0].appendChild(script);
</script>
Examples for installation on well-known frameworks
Check out sample code for installing widgets in different popular frameworks in the repository below.
Updated about 1 month ago